Unit 26 - MODIS ST scripting¶
Let’s start with a simple model for computing LST statistics in Germany area for given period. The raster map is aggregated from input space-time raster dataset modis_cm by t.rast.series. Statistics is computed by r.univar.
t.rast.series input=modis_c output=t_rast_series_out \
where="start_time >= '2023-03-01' and start_time < '2023-04-01'"
r.univar -g --overwrite map=t_rast_series_out
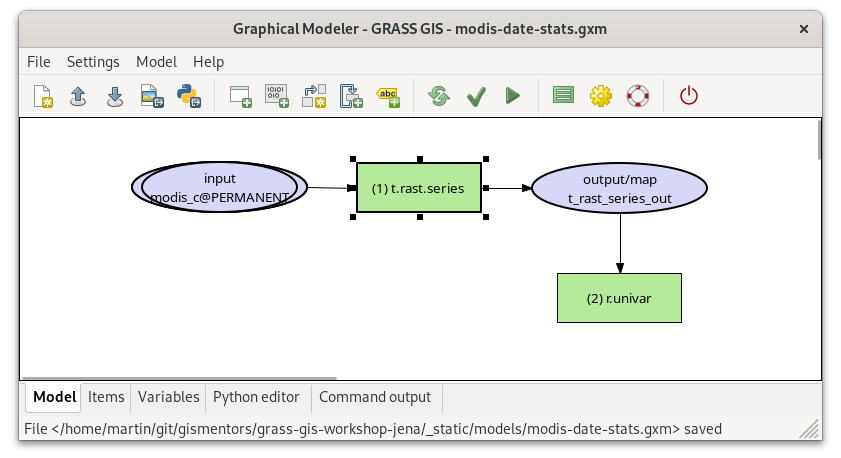
Fig. 120 Sample model to download: modis-date-stats.gxm.¶
In the next step the exported model into Python will be improved:
The option where will be split into two options (18-29,34,60-61): start - Start date and end - End date
New function
check_date()
to validate input dates will be added (35,37,44-47,50-52)Output of r.univar will be parsed and statistics printed (37,72,74-77)
1#!/usr/bin/env python3
2#
3##############################################################################
4#
5# MODULE: modis-date-stats
6#
7# AUTHOR(S): martin
8#
9# PURPOSE: Script generated by wxGUI Graphical Modeler.
10#
11# DATE: Fri Feb 2 18:04:47 2024
12#
13##############################################################################
14
15# %module
16# % description: Computes LST stats for given period (limited to Germany and 2023).
17# %end
18# %option
19# % key: start
20# % description: Start date (eg. 2023-03-01)
21# % type: string
22# % required: yes
23# %end
24# %option
25# % key: end
26# % description: End date (eg. 2023-04-01)
27# % type: string
28# % required: yes
29# %end
30
31import sys
32import os
33import atexit
34from subprocess import PIPE
35from datetime import datetime
36
37from grass.script import parser, fatal, parse_key_val
38from grass.pygrass.modules import Module
39
40def cleanup():
41 Module("g.remove", flags="f", type="raster",
42 name="t_rast_series_out")
43
44def check_date(date_str):
45 d = datetime.strptime(date_str, '%Y-%m-%d')
46 if d.year != 2023:
47 fatal("Only year 2023 allowed")
48
49def main(options, flags):
50 check_date(options['start'])
51 check_date(options['end'])
52
53 Module("t.rast.series",
54 overwrite=True,
55 input="modis_c",
56 method="average",
57 order="start_time",
58 nprocs=1,
59 memory=300,
60 where="start_time >= '{}' and start_time < '{}'".format(
61 options["start"], options["end"]),
62 output="t_rast_series_out",
63 file_limit=1000)
64
65 m = Module("r.univar",
66 flags='g',
67 overwrite=True,
68 map="t_rast_series_out",
69 percentile=90,
70 nprocs=1,
71 separator="pipe",
72 stdout_=PIPE)
73
74 stats = parse_key_val(m.outputs.stdout, val_type=float)
75 print('Min: {0:.1f}'.format(stats['min']))
76 print('Max: {0:.1f}'.format(stats['max']))
77 print('Mean: {0:.1f}'.format(stats['mean']))
78
79 return 0
80
81if __name__ == "__main__":
82 options, flags = parser()
83 atexit.register(cleanup)
84 os.environ["GRASS_OVERWRITE"] = "1"
85 sys.exit(main(options, flags))
Sample script to download: modis-date-stats.py
Example of usage:
modis-date-stats.py start=2023-03-01 end=2023-04-01
Min: -10.6
Max: 11.5
Mean: 3.7