Unit 12 - Script User Interface¶
Standard input¶
In the script prepared in Unit 11 - PyGRASS scripting some tools (r.recode,
r.colors) use hardcoded paths to the input files. See code
below (rules
option):
Module("r.recode",
overwrite=True,
input="ndvi",
output="ndvi_class",
rules="/home/martin/git/gismentors/grass-gis-workshop-jena/_static/models/reclass.txt")
Module("r.colors",
map="ndvi_class_filled",
rules="/home/martin/git/gismentors/grass-gis-workshop-jena/_static/models/colors.txt",
offset=0,
scale=1)
Content of input files can be defined as a string object and transfered
to the command via standard input (stdin_
). See a sample code
below:
Module("r.recode",
overwrite=True,
input="ndvi",
output="ndvi_class",
rules="-",
stdin_="-1:0.1:1\n0.1:0.5:2\n0.5:1:3")
Module("r.colors",
map=options["output"],
rules="-",
stdin_="1 grey\n2 255 255 0\n3 green",
offset=0,
scale=1)
Note that many of GRASS modules allows sending data via standard input
by option value -
(dash). In our case the both commands will be
changed to use rules="-"
syntax.
Task
Define a cleanup routine to remove intermediate data by g.remove tool.
def cleanup():
Module('g.remove', flags='f', name='region_mask', type='vector')
Module('g.remove', flags='f', name='ndvi', type='raster')
Module('g.remove', flags='f', name='ndvi_class', type='raster')
Module('g.remove', flags='f', name='ndvi_class_area', type='raster')
Module('g.remove', flags='f', name='ndvi_class_filled_i', type='raster')
User interface (UI)¶
Let’s improve UI generated by Graphical Modeler in Unit 10 - Python intro.
# %module
# % description: NDVI computation version 3.
# %end
# %option
# % key: voverlay1_ainput
# % description: Name of input vector map (A)
# % required: yes
# % type: string
# % key_desc: name
# % answer: jena_boundary
# %end
# %option
# % key: rreclassarea6_value
# % description: Value option that sets the area size limit (in hectares)
# % required: yes
# % type: double
# % answer: 0.16
# %end
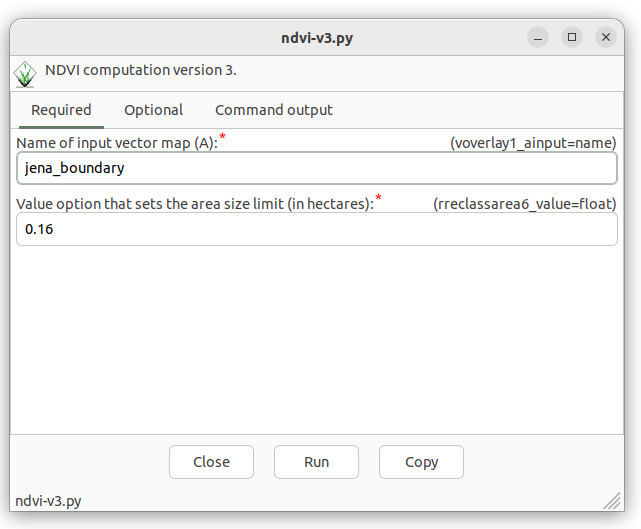
Fig. 73 Generated GUI dialog with voverlay1_ainput
option.¶
Let’s change UI by defining parameters below:
region
: vector map defining a computation region (required)clouds
: vector map with cloud mask features (required)red
: input red channel (required)nir
: input nir channel (required)min_area
: threshold for removing small areas (optional)output
: output vector map (required)
UI definition below.
# %module
# % description: NDVI computation version 4.
# %end
# %option G_OPT_V_INPUT
# % key: region
# % description: Name of input vector region map
# %end
# %option G_OPT_V_INPUT
# % key: clouds
# % description: Name of input vector clouds map
# %end
# %option G_OPT_R_INPUT
# % key: red
# % description: Name of input red channel
# %end
# %option G_OPT_R_INPUT
# % key: nir
# % description: Name of input NIR channel
# %end
# %option
# % key: min_area
# % description: Threshold for removing small areas in m2
# %type: integer
# % answer: 1600
# %end
# %option G_OPT_V_OUTPUT
# %end
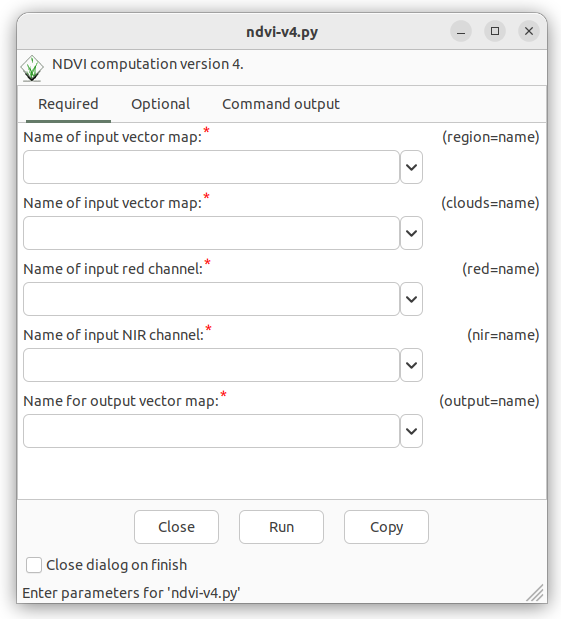
Fig. 74 GUI dialog with input options.¶
In the script input parameters are still hardcoded, eg.
Module("v.overlay",
overwrite=True,
ainput=options["voverlay1_ainput"],
alayer="1",
atype="auto",
binput="MaskFeature",
blayer="1",
btype="area",
operator="not",
output="region_mask",
olayer=['1', '0', '0'],
snap=1e-8)
Input parameters are accesible by options
and flags
objects which are generated by parse()
function.
options, flags = parser()
Options and flags objects are Python dictionaries, where parameters are accessible by keys, see example below.
Module("v.overlay",
overwrite=True,
ainput=options["region"],
alayer="1",
atype="auto",
binput=options["clouds"],
blayer="1",
btype="area",
operator="not",
output="region_mask",
olayer=['1', '0', '0'],
snap=1e-8)
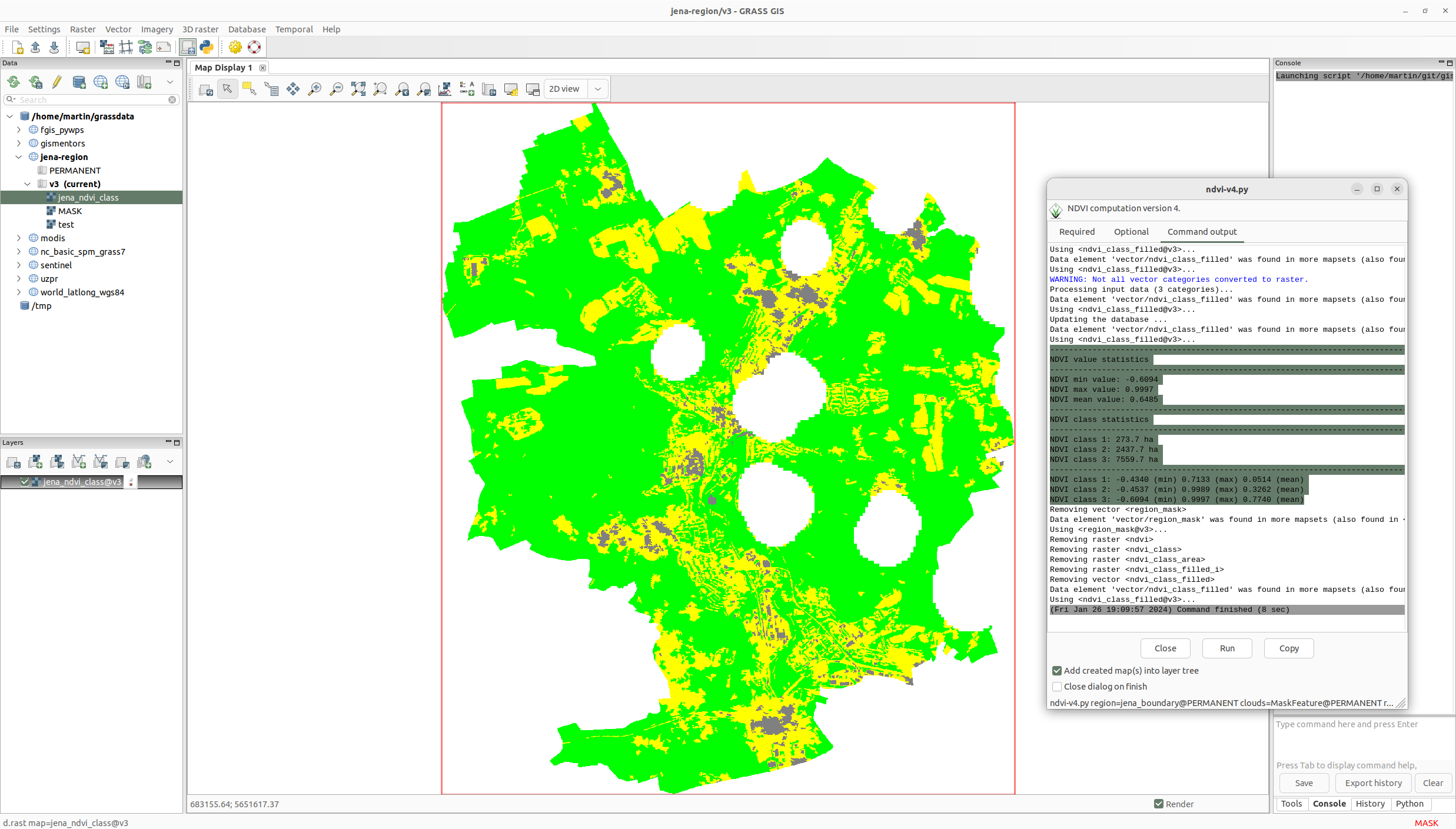
Fig. 75 Improved NDVI script in action.¶
Sample script to download: ndvi-v4.py